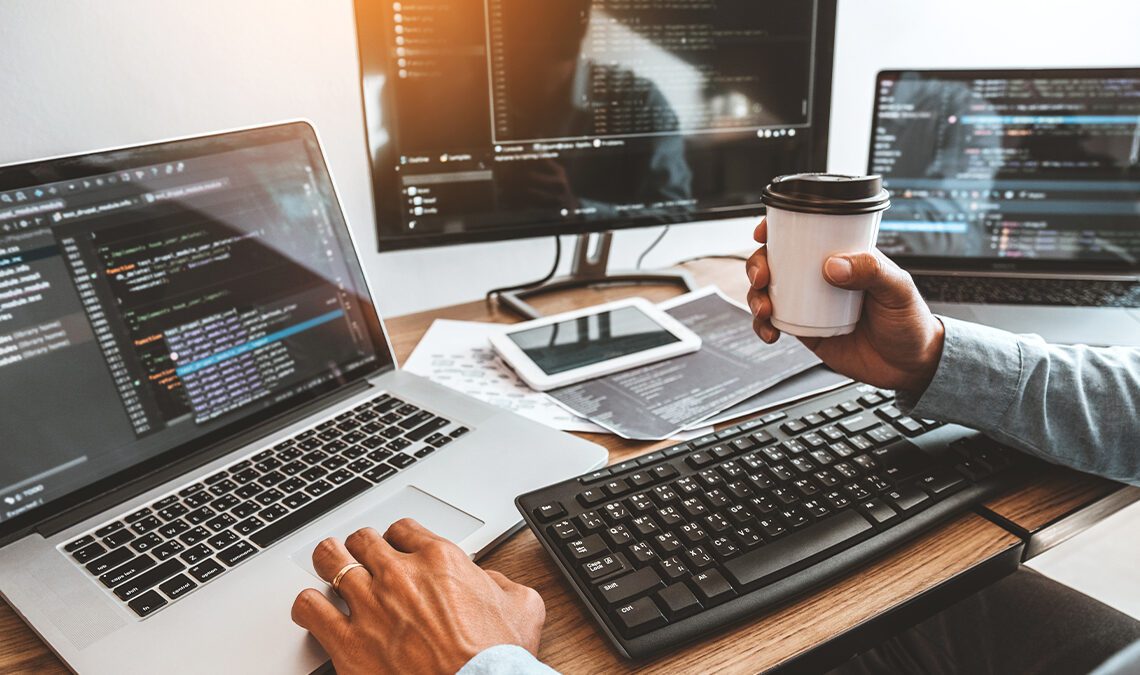
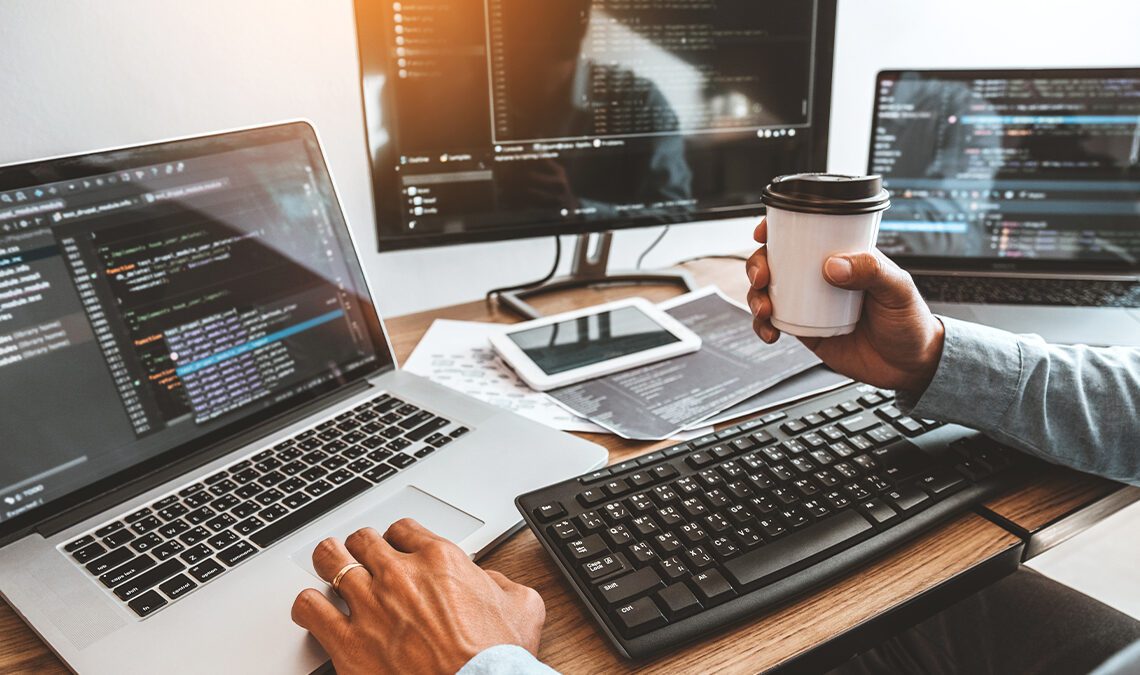
The Airbnb Style Guide has nothing to do with the interior design or physical styling of a vacation rental property, and everything to do with programming and JavaScript style. This article will explain what style guides are and provide simple definitions of the common terms used within the Airbnb Style Guide.
Contents
What Is a Style Guide?
A style guide explains the standards for the way code should be written and organized. It’s made up of rules about the specific way to write code, from line breaks to spaces and other seemingly minor details. (Looking for an interior design style guide, rather than a programming style guide? Check out this article.)
Why Are Style Guides Necessary?
Every code developer has their own way of writing code, which can become problematic when multiple developers are working on the same codebase. With a style guide, developers new to the codebase can easily learn the correct way to write a specific type of code so that it remains cohesive with what has already been written.
A style guide’s goal is to make code seem like it was written by a single person, regardless of how many developers contributed to it. Essentially, a style guide is written to help new developers quickly become comfortable with the codebase so that they can write code that’s simple for other developers to read and understand.
What Is the Airbnb Style Guide?
The Airbnb Style Guide is made up of guidelines and best practices for developers so that they can generate high-quality code. It’s available on Github, which is the world’s largest and most advanced development platform. Millions of companies and developers use Github to build and maintain their software. The style guide for Airbnb is one of the most popular style guides on Github today, and it offers a comprehensive guide to Airbnb code. It’s introduced as “a mostly reasonable approach to JavaScript.”
Definitions of Basic Terms in the Airbnb Style Guide
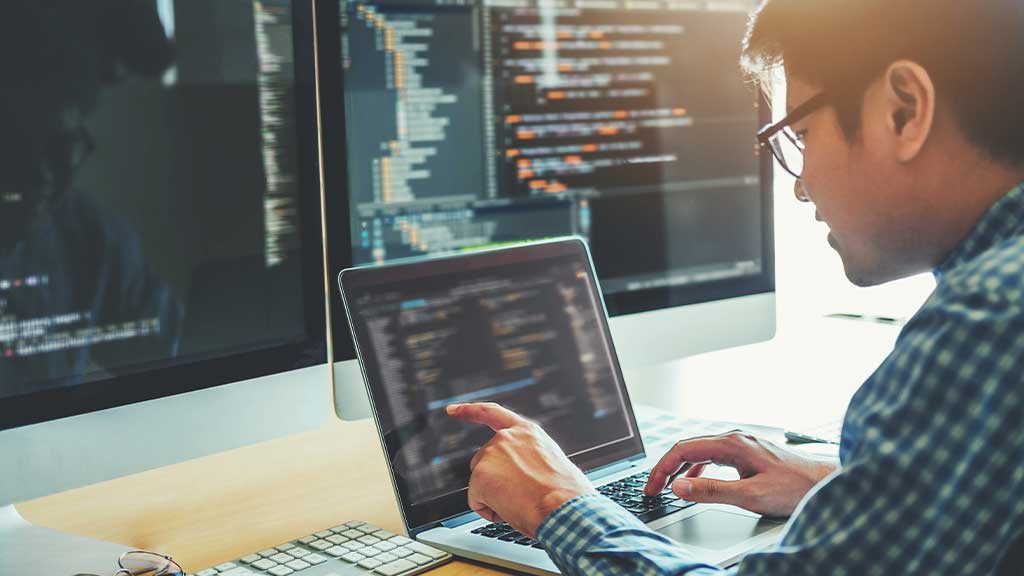
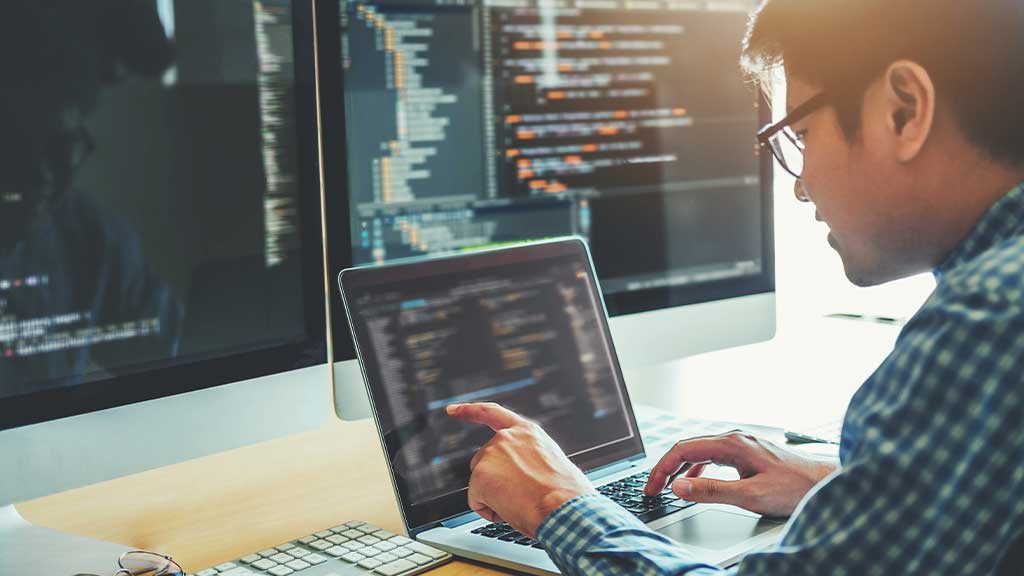
Coding comes with its own language, and when first jumping in, it can be challenging to understand what the Airbnb Style Guide is saying. Here’s a quick overview of some common terms that pop up when discussing the Airbnb Style Guide.
Codebase
A codebase, sometimes written as code base, is the complete collection of human-created programming source code needed to keep the application functional.
JavaScript
JavaScript is a programming language that’s considered more complex than some other languages, such as CSS (Cascading Style Sheets) and HTML (Hypertext Markup Language). Meanwhile, Typescript is a programming language that builds on JavaScript.
JavaScript is text-based and object-oriented, and it’s most often used to create websites that are dynamic and interactive. With JavaScript, developers can create numerous features and functionalities, including countdowns, timers, media players, and image displays.
However, JavaScript isn’t meant to be used on its own; instead, it’s used in conjunction with other languages. For example, the average website needs HTML for its basic structure and CSS for layout and formatting. JavaScript is then added to make the existing web page elements more dynamic. Its main advantages are that it’s extremely popular and widely used, and that it makes websites load more quickly and creates more interactive and exciting components. Plus, JavaScript is simple to implement and can be integrated with many other programming languages. Some of the most popular JavaScript style guides are the Google JavaScript Style Guide and the Airbnb JavaScript Style Guide.
Linting
Linting (along with lint and linter) is another common term in programming that isn’t immediately understandable to those who aren’t familiar with it. However, it’s essential to have a basic understanding of linting for people interested in the Airbnb Style Guide.
Basically, a linter is any tool that inspects code and flags various issues or suspicious usage. Linters can be used with software written in any computer language. They look for bugs, typos, and possible mistakes.
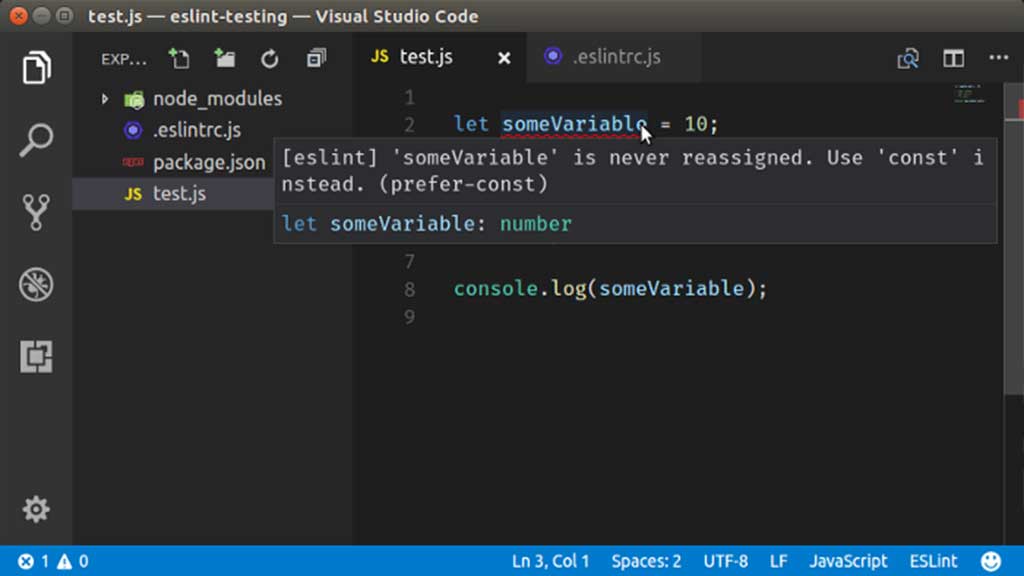
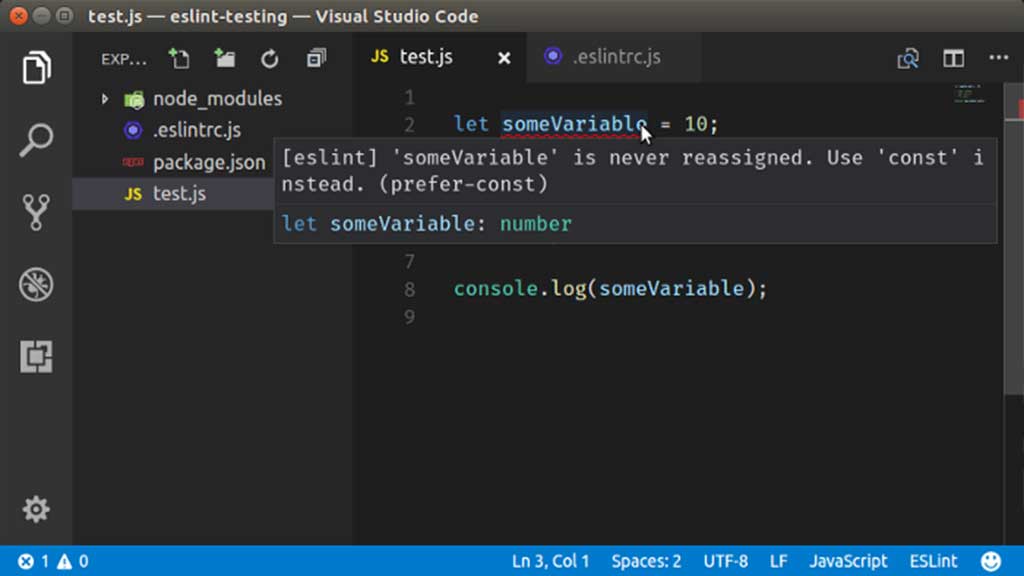
There are a couple of advantages to linting. It provides a style guide for programmers to follow, and it also looks for code that may break or not work properly. Linting makes code cleaner and more consistent, even for developers who are working on their own. Of course, linting is even more important when multiple developers are working together because it helps create a style guide that everyone can adhere to.
ESLint
As people may be able to guess from its name, ESLintis a linter. It allows developers to identify and fix problems in their JavaScript code by statically analyzing it. Most text editors have ESLint built-in, and developers can also run it as part of their continuous integration pipeline. One of the nice things about ESLint is that it can automatically fix many of the problems it identifies. Although ESLint has built-in rules, developers can also write their own rules to work alongside them. This makes ESLint very customizable and useful for any JavaScript project. The Airbnb Style Guide makes multiple references to ESLint and ESLint config for Airbnb.
React
React is a JavaScript library meant for developers to easily build interactive UIs (user interfaces). It efficiently updates and renders the correct components when data changes, making the code more predictable and easier to rid of bugs. React allows developers to build self-managed components that can be put together to create complex user interfaces.
JSX
JSX stands for JavaScript XML, and it is a syntax extension for JavaScript. Syntax extensions are used in code to simplify and coordinate repeated patterns in a program. It makes them more regular and efficient. JSX allows developers to write HTML within JavaScript code in React. The difference between JavaScript and JSX is that JSX is much faster. It’s not mandatory to use JSX, but it does simplify the development of React applications. Airbnb has a React/JSX Style Guide.
Prettier
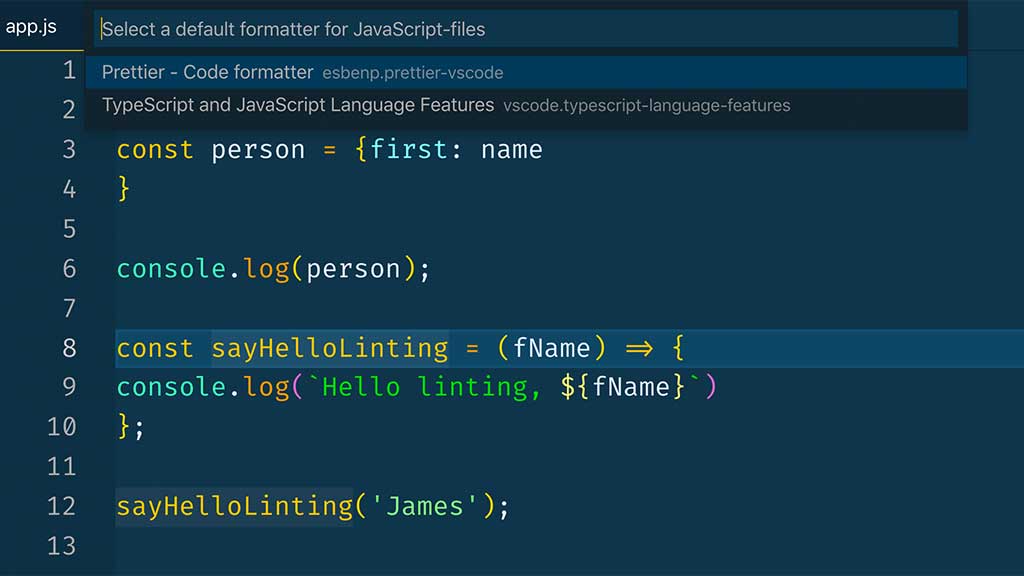
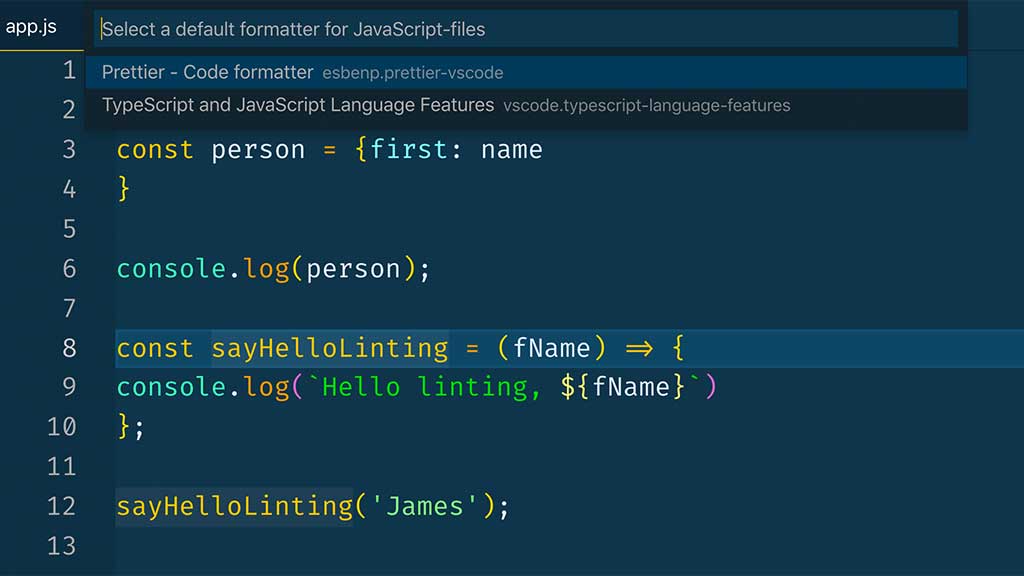
Prettier is an opinionated code formatter (in this context, “opinionated” means that the formatter is built using specific design patterns that make one way of coding significantly easier than others). Prettier integrates with most text editors and can be used with multiple programming languages, including JavaScript, JSX, HTML, and CSS. Using Prettier is commonly considered good practice if a project uses a style guide, such as the Airbnb Style Guide. It assists in creating proper alignment within the code and helps readers understand the code’s overall structure. Prettier enforces consistent styling across the entire codebase.
camelCase and PascalCase
camelCase and PascalCase are naming conventions in programming. camelCase states that each word that makes up a compound word should be capitalized, except for the first word. Meanwhile, PascalCase states all the words’ first letters should be capitalized, including the first word. Since element names can’t contain spaces, camelCase and PascalCase are very useful in programming because they make compound names easy to read.
Config File
A config file (short for “configuration file”) is a text file that contains information needed for a program to operate successfully. Config files are editable and are generally structured to be easily configured by users. They allow users to customize the way they interact with the program, or how the program works with the rest of the system. There’s no standard for how config files should look or how they have to work; this is all left up to the program’s developers.
Overview of the Airbnb Style Guide
The official Github Airbnb Style Guide has 39 topics in its table of contents. The first 15 are briefly outlined in a simplified manner below. For all of the details and examples, be sure to check out the original Airbnb Style Guide. The explanations below do not constitute a comprehensive style guide.
1. Basic Rules
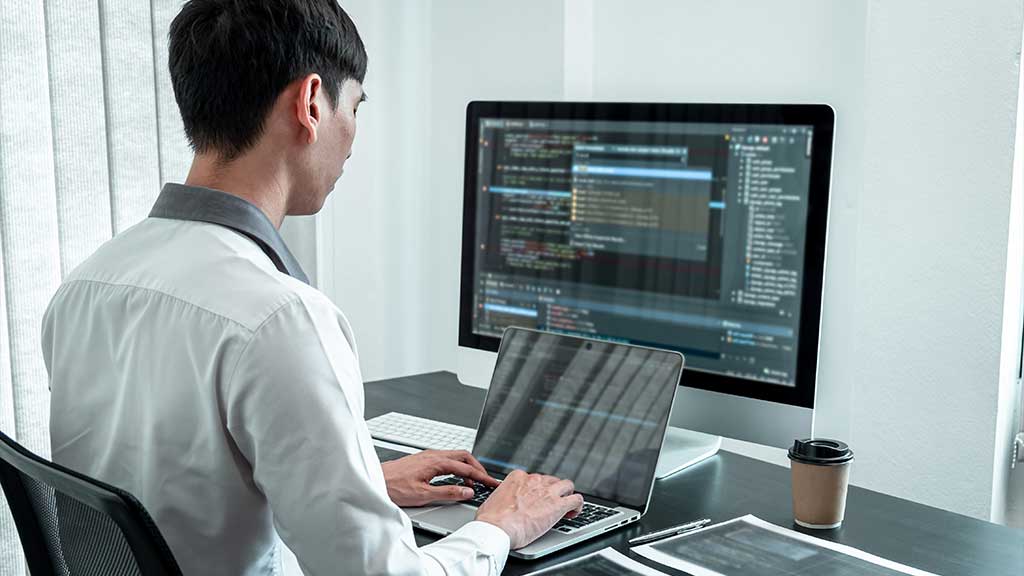
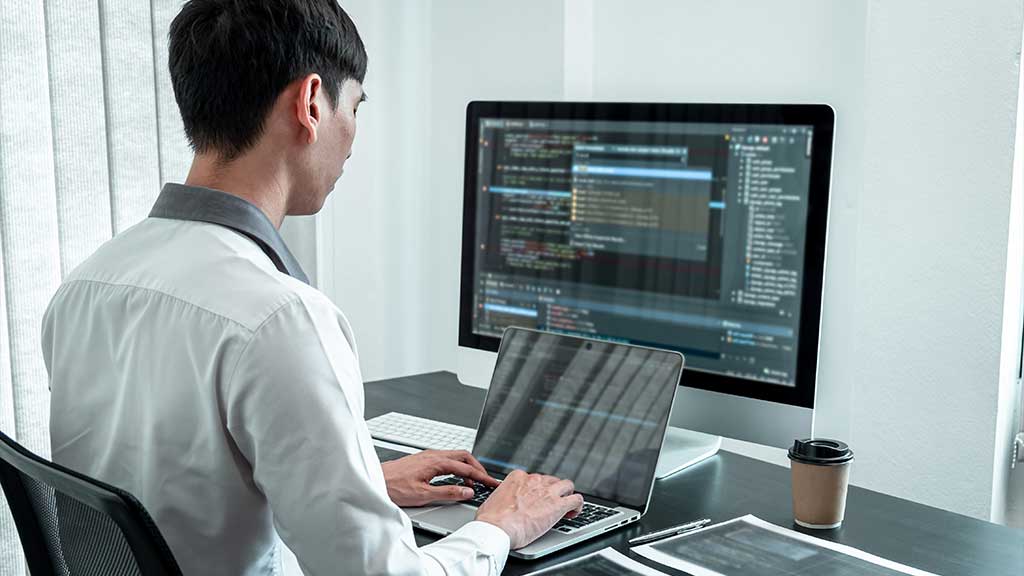
Each file should only have one React component, and developers should only use JSX syntax.
2. Class vs React.createClass vs stateless
Internal state and/or refs should use class extends React.Component, rather than React.createClass.
3. Mixins
Mixins should not be used as they can add unnecessary complexity to the code.
4. Naming
React components should use the .jsx extension; filenames should use PascalCase. PascalCase should be used to name React components, and camelCase should be used for their instances.
5. Declaration
Components should be named by reference.
6. Alignment
The following alignment styles should be used in ESLint for JSX syntax: react/jsx-closing-bracket-location and react/jsx-closing-tag-location.
7. Quotes
JSX attributes should always use double quotes; all other JavaScript should use single quotes.
8. Spacing
Self-closing tags should only include a single space. JSX curly braces should not be padded with spaces.
9. Props
Prop names should be written in camelCase.
10. Refs
Ref callbacks should always be used.
11. Parentheses
When JSX tags span more than one line, they should be wrapped in parentheses.
According to the Airbnb Style Guide, tags that have no children should always be self-closed.
13. Methods
Close over local variables using arrow functions.
14. Ordering
The class extends React.Component should use the following order: optional static methods, constructor, getChildContext, componentWillMount, componentDidMount, componentWillReceiveProps, shouldComponentUpdate, componentWillUpdate, componentDidUpdate, componentWillUnmount, clickHandlers or eventHandlers, getter methods for render, optional render methods, and then render.
15. isMounted
Programmers should not use isMounted, as it is an anti-pattern in the process of being officially deprecated.
List Your Vacation Rental Property on RentalTrader
Interested in listing your vacation rental on sites other than Airbnb? RentalTrader is an excellent option that puts cost-effectiveness at the forefront of the business. There are no guest fees, it’s free to sign up and list your property, and hosts only pay a 4.5% fee on each booking. Get your listing seen by more guests and fill up your calendar with additional stays. List your vacation rental on RentalTrader today!